【入門】Firebase Auth v9でサインアップをハンズオン
Firebase Authentication V9でサインインとサインアップをvueを使ってシンプルにハンズオンします。
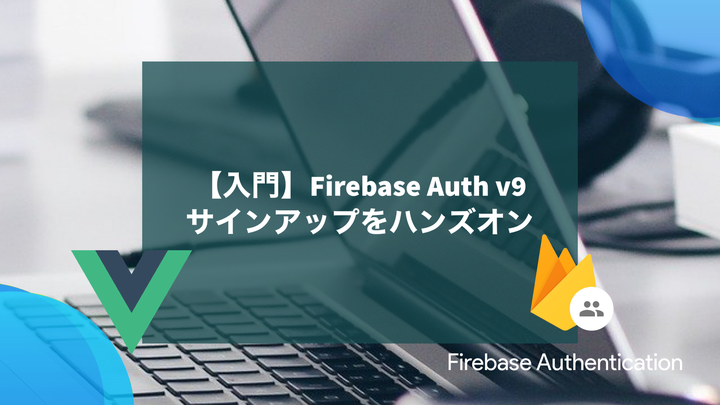
Firebase Authentication V9でサインインとサインアップをvueを使ってシンプルにハンズオンします。 この記事を読むことで、Firebase Authentication v9の基本的な機能サインアップとサインインとサインアウトをvueで実装できるようになります。
目次
firebaseでログイン画面を作るとこまで
今回はvueでハンズオンします。
自分はsdkを触る際に、pythonやrubyのようなスクリプト言語で動かすのが好きなのですが、Firebase Authenticationのようなフロントで触るapiの際はwebpackを使用した環境を作る必要がありました。 ここらへんが素人には導入としては難しかったイメージがあります
今回もvuejsを使ってハンズオンをしていますが、コード自体はできるだけシンプルに書くようにしています。
vueとfirebaseの構成図.png
プロジェクト作成
vue init webpack [プロジェクト名]
サーバー起動
npm run dev
参考 https://firebase.google.com/docs/auth/web/password-auth?hl=ja
アカウント登録
Firebaseの初期化はmainjsで行います。
[src/main.js]
import Vue from 'vue'
import App from './App'
import router from './router'
import { initializeApp } from "firebase/app";
import { getAuth, onAuthStateChanged } from "firebase/auth";
Vue.config.productionTip = false;
let app;
// FirebaseConfigはwebアプリを作成した際に参照できる。
const firebaseConfig = {
apiKey: "",
authDomain: """,
projectId: "",
storageBucket: "",
messagingSenderId: "",
appId: "",
measurementId: ""
};
// Firebaseの初期化
initializeApp(firebaseConfig);
const auth = getAuth();
onAuthStateChanged(auth, user => {
if (!app) {
new Vue({
el: "#app",
router,
components: { App },
template: "<App/>"
});
}
});
サインアップの実装
初期でできているHelloWorld.vueにどんどん書いていきます。 ファイル分けはめんどくさいのでやりません!!!!!!
vueでフォームを作成します。 firebaseのサインアップのメソッドは次のようになります。
createUserWithEmailAndPassword(auth, this.mailaddress, this.password)
全体のファイルはこうなります。 [src/components/HelloWorld.vue]
<template>
<div class="signup">
<h2>サインアップだよ</h2>
<p>メールアドレス
<input type="email" v-model="mailaddress"/></p>
<p>パスワード
<input type="password" v-model="password"/></p>
<button @click="signUp">登録</button>
</div>
</template>
<script>
import { initializeApp } from "firebase/app";
import { getAuth, createUserWithEmailAndPassword } from "firebase/auth";
export default {
name: 'HelloWorld',
data: function () {
return {
mailaddress: null,
password: null,
}
},
methods: {
signUp() {
const auth = getAuth();
createUserWithEmailAndPassword(auth, this.mailaddress, this.password)
.then(userCredential => {
console.log("user created");
})
.catch(error => {
alert(error.message);
console.error(error);
});
}
}
}
</script>
サインインの実装
htmlに以下のコードを追記します
<h2>サインイン</h2>
<p>メールアドレス
<input type="email" v-model="mailaddress_singin"/></p>
<p>パスワード
<input type="password" v-model="password_singin"/></p>
<button @click="signin">サインイン</button>
firebaseのサインイン機能を以下のように追加します。
import { getAuth, createUserWithEmailAndPassword, signInWithEmailAndPassword} from "firebase/auth";
vueにサインインメソッドを追記します
signInWithEmailAndPassword(auth, this.mailaddress_singin, this.password_singin)
全体のファイルはこうなります
[src/components/HelloWorld.vue]
<template>
<div class="signup">
<h2>サインアップだよ</h2>
<p>メールアドレス
<input type="email" v-model="mailaddress"/></p>
<p>パスワード
<input type="password" v-model="password"/></p>
<button @click="signUp">サインアップ</button>
<h2>サインイン</h2>
<p>メールアドレス
<input type="email" v-model="mailaddress_singin"/></p>
<p>パスワード
<input type="password" v-model="password_singin"/></p>
<button @click="signin">サインイン</button>
</div>
</template>
<script>
import { initializeApp } from "firebase/app";
import { getAuth, createUserWithEmailAndPassword, signInWithEmailAndPassword} from "firebase/auth";
export default {
name: 'HelloWorld',
data: function () {
return {
mailaddress: null,
password: null,
mailaddress_singin: null,
password_singin: null,
}
},
methods: {
signUp() {
const auth = getAuth();
createUserWithEmailAndPassword(auth, this.mailaddress, this.password)
.then(userCredential => {
console.log("user created");
})
.catch(error => {
alert(error.message);
console.error(error);
});
},
signin(){
const auth = getAuth();
signInWithEmailAndPassword(auth, this.mailaddress_singin, this.password_singin)
.then(userCredential => {
console.log("singin");
})
.catch(error => {
alert(error.message);
console.error(error);
});
}
}
}
</script>
サインアウトの実装
サインアウト用のボタンを追加します
<button @click="signout_botton">サインアウト</button>
firebaseのサインアウトメソッドを追加します。
import { getAuth, createUserWithEmailAndPassword, signInWithEmailAndPassword, signOut} from "firebase/auth";
サインアウトの処理を追記します
signout_botton(){
const auth = getAuth();
signOut(auth).then(() => {
console.log("singout");
})
.catch((error) => {
alert(error.message);
console.error(error);
});
}
全体のファイルはこうなります
<template>
<div class="signup">
<h2>サインアップだよ</h2>
<p>メールアドレス
<input type="email" v-model="mailaddress"/></p>
<p>パスワード
<input type="password" v-model="password"/></p>
<button @click="signUp">サインアップ</button>
<h2>サインイン</h2>
<p>メールアドレス
<input type="email" v-model="mailaddresssingin"/></p>
<p>パスワード
<input type="password" v-model="passwordsingin"/></p>
<button @click="signin">サインイン</button>
<button @click="signout_botton">サインアウト</button>
</div>
</template>
<script>
import { initializeApp } from "firebase/app";
import { getAuth, createUserWithEmailAndPassword, signInWithEmailAndPassword, signOut} from "firebase/auth";
export default {
name: 'HelloWorld',
data: function () {
return {
mailaddress: null,
password: null,
mailaddresssingin: null,
passwordsingin: null,
}
},
methods: {
signUp() {
const auth = getAuth();
createUserWithEmailAndPassword(auth, this.mailaddress, this.password)
.then(userCredential => {
console.log("user created");
})
.catch(error => {
alert(error.message);
console.error(error);
});
},
signin(){
const auth = getAuth();
signInWithEmailAndPassword(auth, this.mailaddresssingin, this.passwordsingin)
.then(userCredential => {
console.log("singin");
})
.catch(error => {
alert(error.message);
console.error(error);
});
},
signout_botton(){
const auth = getAuth();
signOut(auth).then(() => {
console.log("singout");
})
.catch((error) => {
alert(error.message);
console.error(error);
});
}
}
}
</script>
まとめ
Firebase Authentication v9でサインアップとサインインとサインアウトをしました。 v8の記事だらけで非常にキャッチアップがしにくい状況ではありますが、認証周りはマイクロサービスの一番のハードルなのでクリアしたいですね。